译(二十七)-Python对列表内元素计数
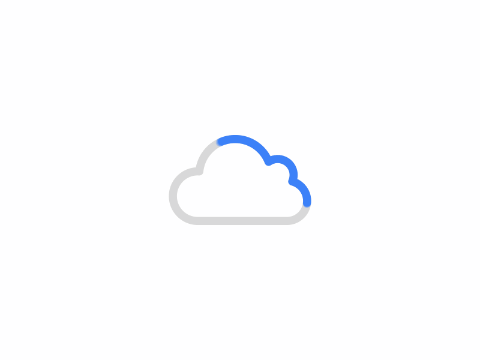
如有翻译问题欢迎评论指出,谢谢。
Python如何对列表内元素进行计数
-
weakish asked:
- 给定一个元素,怎么在Python里计算列表中该元素出现的次数?
-
Answers:
- Łukasz - vote: 2187
-
如果只需要一个元素的计数,可以用
count
函数: -
>>> [1, 2, 3, 4, 1, 4, 1].count(1)
3
-
关于
count
的性能需要注意: -
不要在多元素的计数中使用
count
。 -
在循环中调用
count
时,会在每次的调用中单独遍历列表,这使得其性能降低非常多。 -
如果想要计算所有元素出现的次数,或者只是其中一部分元素,使用
counter
,用法可参考其他用户的答案。 - user52028778 - vote: 2100
-
如果在Python2.7或3.x中,可以使用
Counter
对列表中每个元素进行计数。 -
>>> from collections import Counter
>>> z = ['blue', 'red', 'blue', 'yellow', 'blue', 'red']
>>> Counter(z)
Counter({'blue': 3, 'red': 2, 'yellow': 1})
- user2314737 - vote: 316
- 计数列表中元素出现的个数
-
使用
count()
可以对列表中的某个元素出现的次数进行计数 -
>>> l = [a,b,b]
>>> l.count(a)
1
>>> l.count(b)
2
- 计数列表中所有元素出现的次数,被称为列表统计,或创建列表计数器。
-
用
count()
对所有元素进行计数 -
使用列表推导式和
count()
函数可以计算l
中元素的出现次数。 -
[[x,l.count(x)] for x in set(l)]
-
(类似地,也可以用字典
dict((x,l.count(x)) for x in set(l))
) - 例如:
-
>>> l = [a,b,b]
>>> [[x,l.count(x)] for x in set(l)]
[['a', 1], ['b', 2]]
>>> dict((x,l.count(x)) for x in set(l))
{'a': 1, 'b': 2}
-
用
counter()
对所有元素进行计数 -
还有一种选择,使用
collections
库中更快的counter
类。 -
Counter(l)
- 例如
-
>>> l = [a,b,b]
>>> from collections import Counter
>>> Counter(l)
Counter({'b': 2, 'a': 1})
-
counter
性能如何? -
我研究了一下
counter
在列表统计中的效率。我用两个方法来比较,结果表明counter
的效率比count
快很多。 - 代码如下:
from __future__ import print_function import timeit t1=timeit.Timer('Counter(l)', \ 'import random;import string;from collections import Counter;n=1000;l=[random.choice(string.ascii_letters) for x in range(n)]' ) t2=timeit.Timer('[[x,l.count(x)] for x in set(l)]', 'import random;import string;n=1000;l=[random.choice(string.ascii_letters) for x in range(n)]' ) print("Counter(): ", t1.repeat(repeat=3,number=10000)) print("count(): ", t2.repeat(repeat=3,number=10000)
- 输出如下:
-
Counter(): [0.46062711701961234, 0.4022796869976446, 0.3974247490405105]
count(): [7.779430688009597, 7.962715800967999, 8.420845870045014]
How can I count the occurrences of a list item?
-
weakish asked:
- Given an item, how can I count its occurrences in a list in Python?
给定一个元素,怎么在Python里计算列表中该元素出现的次数?
- Given an item, how can I count its occurrences in a list in Python?
-
Answers:
- Łukasz - vote: 2187
-
If you only want one item\'s count, use the
count
method:
如果只需要一个元素的计数,可以用count
函数: -
>>> [1, 2, 3, 4, 1, 4, 1].count(1)
3
-
Important Note regarding count performance
关于count
的性能需要注意: -
Don\'t use this if you want to count multiple items.
不要在多元素的计数中使用count
。 -
Calling
count
in a loop requires a separate pass over the list for everycount
call, which can be catastrophic for performance.
在循环中调用count
时,会在每次的调用中单独遍历列表,这使得其性能降低非常多。 -
If you want to count all items, or even just multiple items, use
Counter
, as explained in the other answers.
如果想要计算所有元素出现的次数,或者只是其中一部分元素,使用counter
,用法可参考其他用户的答案。 - user52028778 - vote: 2100
-
Use
Counter
if you are using Python 2.7 or 3.x and you want the number of occurrences for each element:
如果在Python2.7或3.x中,可以使用Counter
对列表中每个元素进行计数。 -
>>> from collections import Counter
>>> z = ['blue', 'red', 'blue', 'yellow', 'blue', 'red']
>>> Counter(z)
Counter({'blue': 3, 'red': 2, 'yellow': 1})
- user2314737 - vote: 316
-
Counting the occurrences of one item in a list
计数列表中元素出现的个数 -
For counting the occurrences of just one list item you can use
count()
使用count()
可以对列表中的某个元素出现的次数进行计数 -
>>> l = [a,b,b]
>>> l.count(a)
1
>>> l.count(b)
2
-
Counting the occurrences of all items in a list is also known as tallying a list, or creating a tally counter.
计数列表中所有元素出现的次数,被称为列表统计,或创建列表计数器。 -
Counting all items with count()
用count()
对所有元素进行计数 -
To count the occurrences of items in
l
one can simply use a list comprehension and thecount()
method
使用列表推导式和count()
函数可以计算l
中元素的出现次数。 -
[[x,l.count(x)] for x in set(l)]
-
(or similarly with a dictionary
dict((x,l.count(x)) for x in set(l))
)
(类似地,也可以用字典dict((x,l.count(x)) for x in set(l))
) -
Example:
例如: -
>>> l = [a,b,b]
>>> [[x,l.count(x)] for x in set(l)]
[['a', 1], ['b', 2]]
>>> dict((x,l.count(x)) for x in set(l))
{'a': 1, 'b': 2}
-
Counting all items with Counter()
用counter()
对所有元素进行计数 -
Alternatively, there\'s the faster
Counter
class from thecollections
library
还有一种选择,使用collections
库中更快的counter
类。 -
Counter(l)
-
Example:
例如 -
>>> l = [a,b,b]
>>> from collections import Counter
>>> Counter(l)
Counter({'b': 2, 'a': 1})
-
How much faster is Counter?
counter
性能如何? -
I checked how much faster
Counter
is for tallying lists. I tried both methods out with a few values ofn
and it appears thatCounter
is faster by a constant factor of approximately 2.
我研究了一下counter
在列表统计中的效率。我用两个方法来比较,结果表明counter
的效率比count
快很多。 -
Here is the script I used:
代码如下: from __future__ import print_function import timeit t1=timeit.Timer('Counter(l)', \ 'import random;import string;from collections import Counter;n=1000;l=[random.choice(string.ascii_letters) for x in range(n)]' ) t2=timeit.Timer('[[x,l.count(x)] for x in set(l)]', 'import random;import string;n=1000;l=[random.choice(string.ascii_letters) for x in range(n)]' ) print("Counter(): ", t1.repeat(repeat=3,number=10000)) print("count(): ", t2.repeat(repeat=3,number=10000)
-
And the output:
输出如下: -
Counter(): [0.46062711701961234, 0.4022796869976446, 0.3974247490405105]
count(): [7.779430688009597, 7.962715800967999, 8.420845870045014]
共有 0 条评论